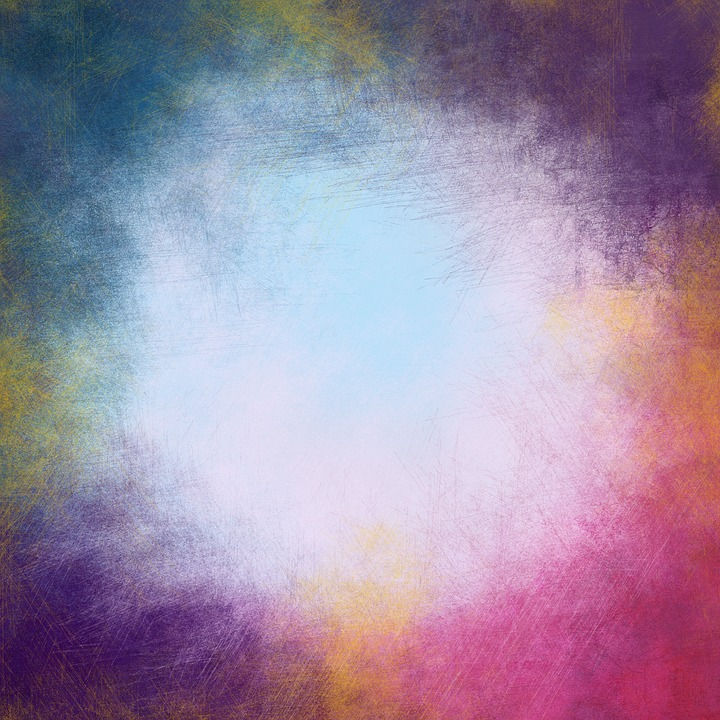
Research and Development
Elizabeth Hargrove
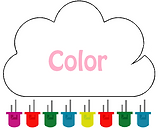
Development: Coding
Now that I know how to work sensors and LED's, it's time for me to make the code behind my final product.
My Weather Station Code
First Code, before I changed my sensor:
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
// named constant for the pin the sensor is connected to
const int sensorPin = A0;
const float baselineTemp = 22.0;
// room temperature in Celcius
/*
Connect one end of the photocell to 5V, the other end to Analog 1.
Then connect one end of a 10K resistor from Analog 1 to ground
Connect LED from pin 11 through a resistor to ground */
int photocellPin = 1; // the cell and 10K pulldown are connected to a0
int photocellReading; // the analog reading from the sensor divider
int LEDpin = 11; // connect Red LED to pin 11 (PWM pin)
int LEDbrightness; //
void setup(void) {
// We'll send debugging information via the Serial monitor
Serial.begin(9600);
// set the LED pins as outputs
// the for() loop saves some extra coding
for(int pinNumber = 2; pinNumber<5; pinNumber++){
pinMode(pinNumber,OUTPUT);
digitalWrite(pinNumber, LOW);
}
}
void loop(){
// read the value on AnalogIn pin 0
// and store it in a variable
int sensorVal = analogRead(sensorPin);
// send the 10-bit sensor value out the serial port
Serial.print("sensor Value: ");
Serial.print(sensorVal);
// convert the ADC reading to voltage
float voltage = (sensorVal/1024.0) * 5.0;
// Send the voltage level out the Serial port
Serial.print(", Volts: ");
Serial.print(voltage);
// convert the voltage to temperature in degrees C
// the sensor changes 10 mV per degree
// the datasheet says there's a 500 mV offset
// ((volatge - 500mV) times 100)
Serial.print(", degrees C: ");
float temperature = (voltage - .5) * 100;
Serial.println(temperature);
if(temperature < baselineTemp){
digitalWrite(2, LOW);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
} // if the temperature rises 2-4 degrees, turn an LED on
else if(temperature >= baselineTemp+2 && temperature < baselineTemp+4){
digitalWrite(2, HIGH);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
} // if the temperature rises 4-6 degrees, turn a second LED on
else if(temperature >= baselineTemp+4 && temperature < baselineTemp+6){
digitalWrite(2, HIGH);
digitalWrite(3, HIGH);
digitalWrite(4, LOW);
} // if the temperature rises more than 6 degrees, turn all LEDs on
else if(temperature >= baselineTemp+6){
digitalWrite(2, HIGH);
digitalWrite(3, HIGH);
digitalWrite(4, HIGH);
}
/// Photocell Stuff
photocellReading = analogRead(photocellPin);
Serial.print("Analog reading = ");
Serial.println(photocellReading); // the raw analog reading
// LED gets brighter the darker it is at the sensor
// that means we have to -invert- the reading from 0-1023 back to 1023-0
photocellReading = photocellReading - 1023;
//now we have to map 0-1023 to 0-255 since thats the range analogWrite uses
LEDbrightness = map(photocellReading, 0, 1023, 0, 255);
analogWrite(LEDpin, LEDbrightness);
delay(100);
}

Code for Final Prototype!!
#include <stdint.h>
#include "SparkFunBME280.h"
#include "Wire.h"
#include "SPI.h"
const float baselineTemp = 22.0;
const float baselineHum = 55;
BME280 mySensor;
float TemVar = 0.0;
float PreVar = 0.0;
float HumVar = 0.0;
int Templow = 10;
int Tempgood = 11;
int Temphigh = 12;
int humlow = 4;
int humgood = 5;
int humhigh = 6;
int prepin = 7;
int photocellPin = A0; // the cell and 10K pulldown are connected to a0
int photocellReading; // the analog reading from the sensor divider
int LEDpin = 9;// connect Red LED to pin 11 (PWM pin)
int LEDbrightness; //
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
mySensor.begin();
pinMode(Templow, OUTPUT);
pinMode(Tempgood, OUTPUT);
pinMode(Temphigh, OUTPUT);
pinMode(LEDpin, OUTPUT);
pinMode(humlow, OUTPUT);
pinMode(humgood, OUTPUT);
pinMode(humhigh, OUTPUT);
}
void loop() {
// put your main code here, to run repeatedly:
TemVar = mySensor.readTempC();
Serial.print("Degrees C: ");
Serial.println(TemVar);
delay(100);
if(TemVar < baselineTemp){
digitalWrite(10, HIGH);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
} // if the temperature rises 0-6 degrees, turn an LED on
else if(TemVar >= baselineTemp && TemVar < baselineTemp+6){
digitalWrite(10, HIGH);
digitalWrite(11, HIGH);
digitalWrite(12, LOW);
} // if the temperature rises more than 6 degrees, turn all LEDs on
else if(TemVar >= baselineTemp+6){
digitalWrite(10, HIGH);
digitalWrite(11, LOW);
digitalWrite(12, LOW);
}
PreVar = mySensor.readFloatPressure();
Serial.print("Pressure: ");
Serial.println(PreVar);
delay(100);
if(PreVar < 65000.00);{
digitalWrite(7, HIGH);
}
if(PreVar > 68000.00);{
digitalWrite(7, HIGH);
}
HumVar = mySensor.readFloatHumidity();
Serial.print("Humidity: ");
Serial.println(HumVar);
delay(100);
if(HumVar < baselineHum){
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
digitalWrite(6, LOW);
} // if the Humidity rises 0-19%, turn an LED on
else if(HumVar >= baselineHum && HumVar < baselineHum+20){
digitalWrite(4, HIGH);
digitalWrite(5, HIGH);
digitalWrite(6, LOW);
} // if the Humidity rises more than 20%, turn all LEDs on
else if(HumVar >= baselineHum+20){
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
digitalWrite(6, LOW);
}
/// Photocell Stuff
photocellReading = analogRead(photocellPin);
Serial.print("Analog photo reading = ");
Serial.println(photocellReading); // the raw analog reading
// LED gets brighter the darker it is at the sensor
// that means we have to -invert- the reading from 0-1023 back to 1023-0
photocellReading = photocellReading - 1023;
//now we have to map 0-1023 to 0-255 since thats the range analogWrite uses
LEDbrightness = map(photocellReading, 0, 1023, 0, 255);
analogWrite(LEDpin, LEDbrightness);
delay(100);
}